Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
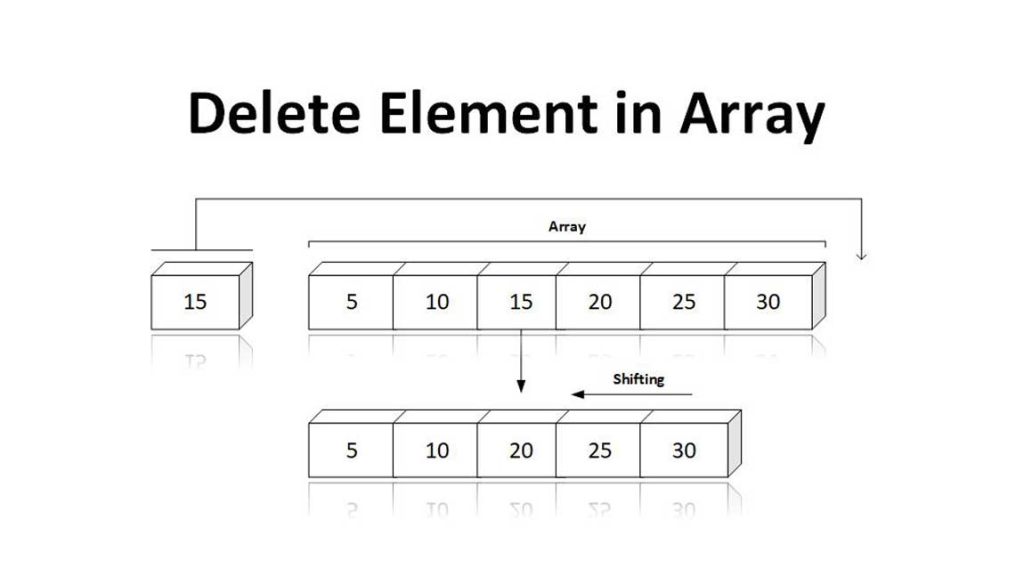
One of the most common tasks in PHP is deleting an element from an array. We will introduce methods to delete an element from an array in PHP.
- Using
unset()
function - Using
array_splice()
function - Using
array_diff()
function
Using the unset() Function
Use unset()
Function to Delete an Element From an Array in PHP
The built-in function unset()
is used to delete the value stored in a variable. It is only applicable to the local variables. It does not reflect its behavior on global variables. We can use this function to delete an element from an array.
unset($variableName);
It has a single mandatory parameter. The variable whose value we wish to delete is passed as a parameter to this function.
Example:-
<?php
//Declare the array
$nicname = array(
"Vijay",
"Amit",
"Rahul",
"Dharmendra",
"Roshan");
unset($nicname[2]);
echo "The array is:\n";
print_r($nicname);
?>
This function can delete one value at a time. The name of the array along with the element index ($nicname[2]
) is passed as a parameter. This function does not change the index values. The index values remain the same as they were before.
Output:
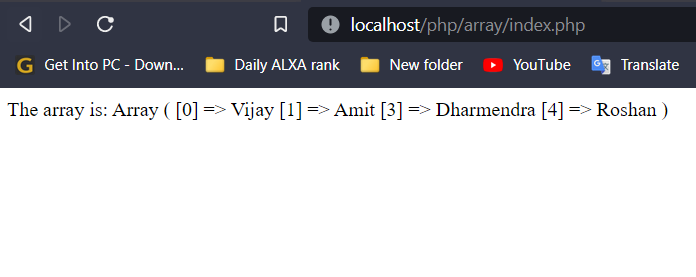
As you could see, the index 1
is missing after we apply the unset
function.
Use array_splice()
Function to Delete an Element From an Array in PHP
The function array_splice()
is used to add elements to an array or delete elements from an array.
array_splice($arrayName, $startingIndex, $numOfElements, $array2Name);
It has four parameters.
$arrayName
is a mandatory parameter. It is the array whose elements will be deleted.$startingIndex
is the index of the element we wish to delete.$numOfElements
is the number of elements we want to delete from the starting index.$array2Name
is an array of elements we want to add.
<?php
//Declare the array
$nicname = array(
"Vijay",
"Amit",
"Dharmu",
"Roshan",
"Dilip",
"Rahul",
"Dhyanchand",
"Shohan");
array_splice($nicname, 4, 3);
echo "The array is:\n";
print_r($nicname);
?>
The array $nicname
is passed as a parameter to this function along with the starting index 4 and the number of elements we want to delete-3. In this way, we can delete multiple elements from an array.
Output:-
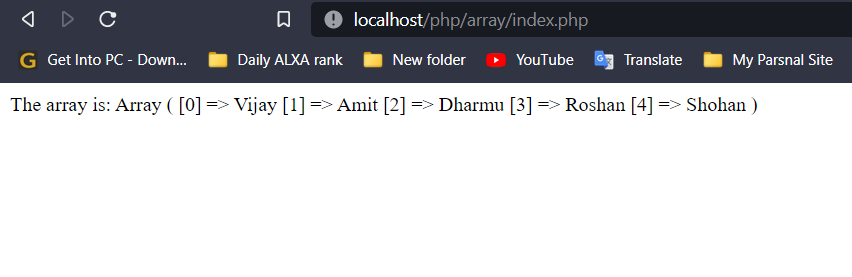
Different from unset
, array_splice
the function will automatically reindex the keys.
Daisy
has the new index as 4 but not the original index – 7, after we delete three elements before it.
Use array_diff()
Function to Delete an Element From an Array in PHP
The built-in function array_diff()
 finds the difference between two or more arrays
. It can be used to delete multiple values from an array without affecting their indexes.
array_diff($array1, $array2, $array3, ... , $arrayN);
It takes N number of arrays
as the parameters. It compares the first array with all other arrays and returns an array that contains all the elements of the first array that are not present in other arrays.
<?php
//Declare the array
$nicname = array(
"Vijay",
"Amit",
"Dharmu",
"Roshan",
"Rahul",
"Devanand",
"Ramayan",
"Dillyan");
$nicname = array_diff($nicname, array("Vijay","Amit"));
echo "The array is:\n";
print_r($nicname);
?>
Here, the first array that we have passed is $nicname
and the second array contains the elements that we want to delete from $nicname
. This function does not change the indexes of the elements of the array.
Output:-
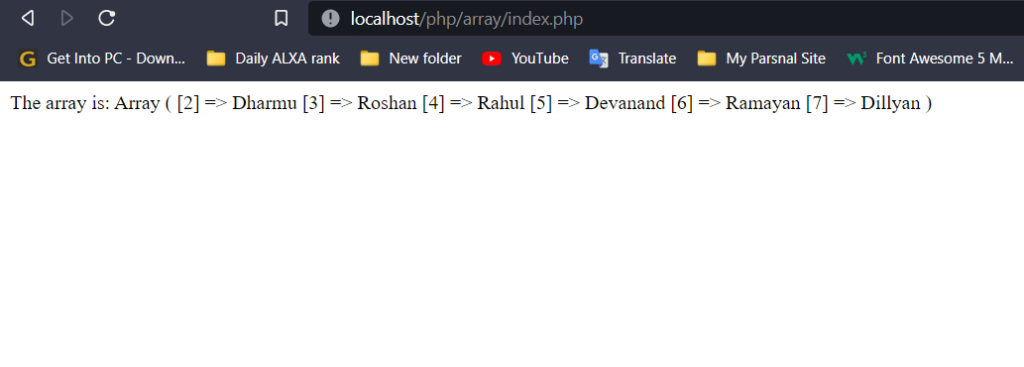
Leave a Reply