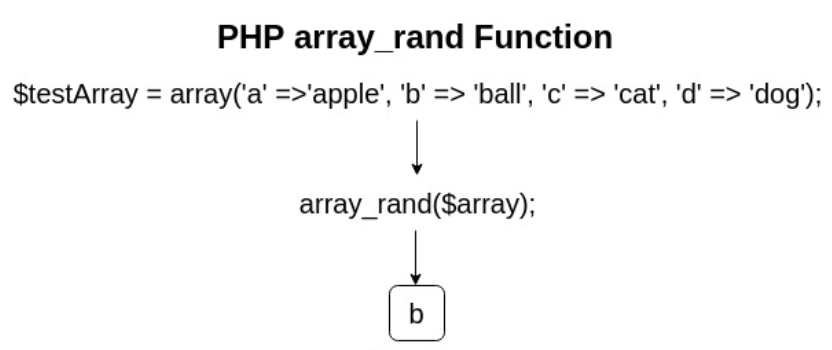
There are two types of getting a random value from a PHP array.
- array_rand() function
- shuffle() function
array_rand() function
When working with PHP, sometimes you need to get random values from an array. This can be easily accomplished with the array_rand() function. We will show in this post several methods that can be used to get random items from an array.
The array_rand() function is used to get a random value from an array. This function accepts an array as a parameter and returns a random key from the array.
Syntax:-
array_rand($array, $number_of_items)
Parameters
The array_rand() function takes two parameters. One is the array from which you want to get the random key.
$array: [Required] This is a required parameter that array_rand() takes.
$number_of_items: [Optional] This is the int value that shows how many random keys you want to get from the array. Its value is set to 1 by default.
Example:-
<?php
$my_arr = ["Vijay", "Amit", "Dharmu", "Rahul", "Ravi"];
$rand_index = array_rand($my_arr);
echo $my_arr[$rand_index];
?>
Output:-
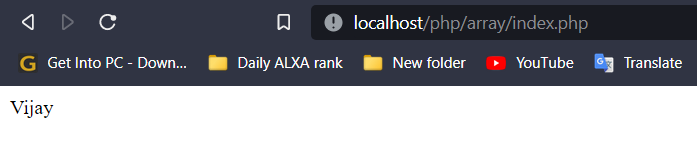
The output will be different each time you execute the above code. If you want to get more than 1 random key from the array you can use the below code example.
Example:
<?php
$my_arr = ["Vijay", "Amit", "Dharmu", "Rahul", "Ravi"];
$rand_index = array_rand($my_arr, 2);
echo $my_arr[$rand_index[0]];
echo $my_arr[$rand_index[1]];
?>
Output:-
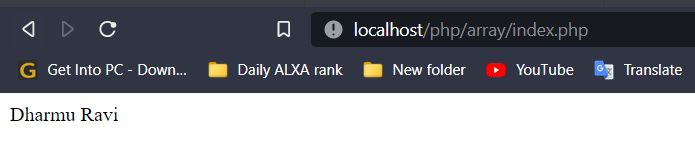
shuffle() function
If you’re looking for a quick and easy way to get a random item from an array using PHP, the shuffle() function is the way to go. This function will randomly shuffle the items in an array, making it easy to get a random item.
Syntax:-
shuffle($arr)[0];
Example:-
<?php
$my_arr = [
"a" => 100,
"b" => 200,
"c" => 300,
"4" => 400
];
shuffle($my_arr);
echo $my_arr[0];
?>
Output
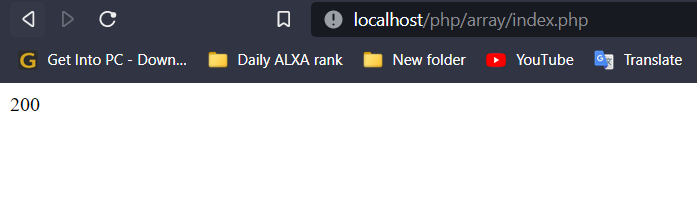