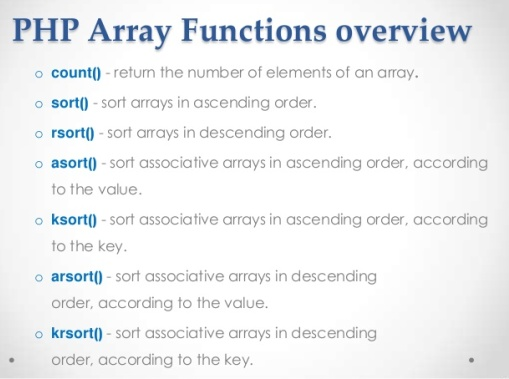
What is Array?
The array is a collection of items. The array is used for data types. The array is a special type of data structure that stores fixed-size data collection. We can store any amount of data in the array and use it easily. It also works like a database in which we can store the data. You can store it and use it. The array is a collection in which a lot of data can be stored. Array removes the complexity of the data. Many data problems can be removed by using PHP Array. The array() function is used to create an array. PHP array() function can contain (store, collect) many values.
Syntax:-
array("value1", "value2 ", "value3");
Example 1:-
<?php
$fruits = array("Banana", "Mango ", "Orange");
echo "I like " . $fruits[0] . ", " . $fruits[1] . " and " . $fruits[2] . ".";
?>
Output:-
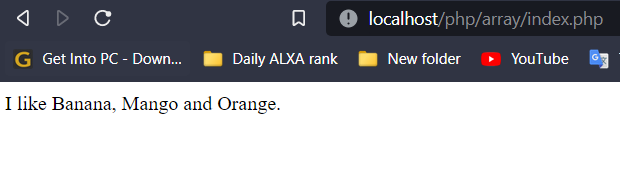
1. array_merge() function
The “array_merge” function provides the easiest way to merge a list of arrays. It can take as many parameters as you give to it and it will merge all of them into a single one and return back to you.
Example:-
<?php
$myarray1 = array(1,2,3);
$myarray2 = array(4,5,6);
$myarray3 = array(7,8,9);
$single_merged_array = array_merge($myarray1,$myarray2,$myarray3);
print_r($single_merged_array);
?>
Output:-
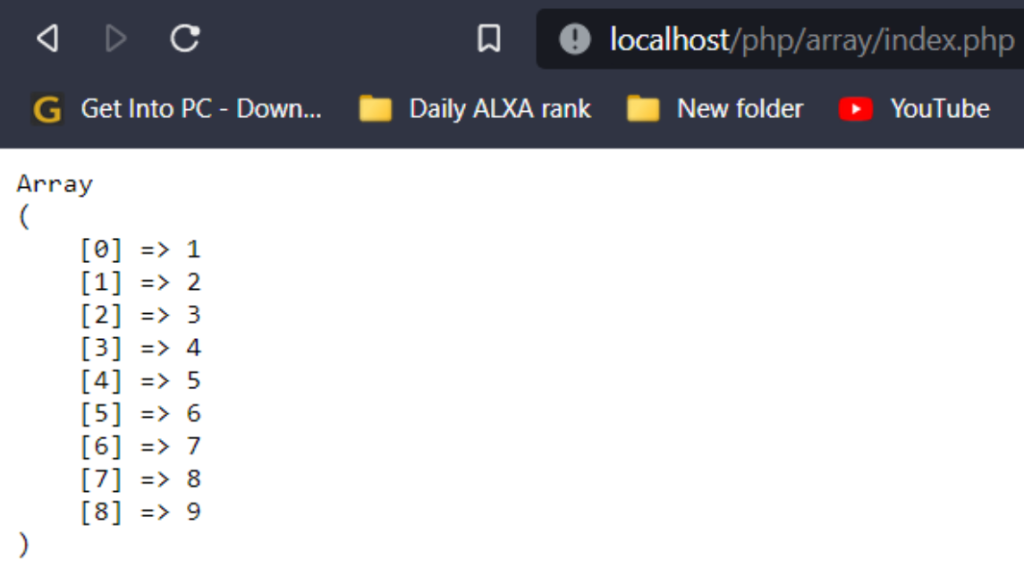
2. Sorting Arrays In PHP:
There are two ways to sort elements of an array. If you want a simple default ascending order sorting with non-object array elements, then you can go for the “sort” function. You just pass the array as a parameter and it will sort the array and returns true if successful.
Example:-
<?php
$simple_array = array("dbc","bcd","abc");
if(sort($simple_array)){
echo "<pre>";
print_r($simple_array);
echo "</pre>";
}
?>
Output:-
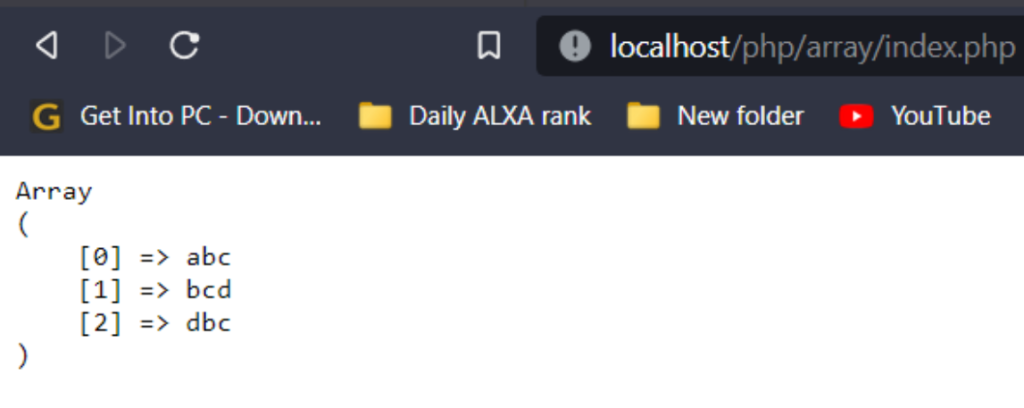
3. array_diff() function
If you are trying to exclude a few elements from an array that is common to another array’s elements, then “array_diff” is the perfect function for you.
Example:-
<?php
$myarray1 = array(1,2,3,4,-1,-2);
$myarray2 = array(-1,-2);
$filtered_array = array_diff($myarray1,$myarray2);
echo "<pre>";
print_r($filtered_array);
echo "</pre>";
?>
Output:-
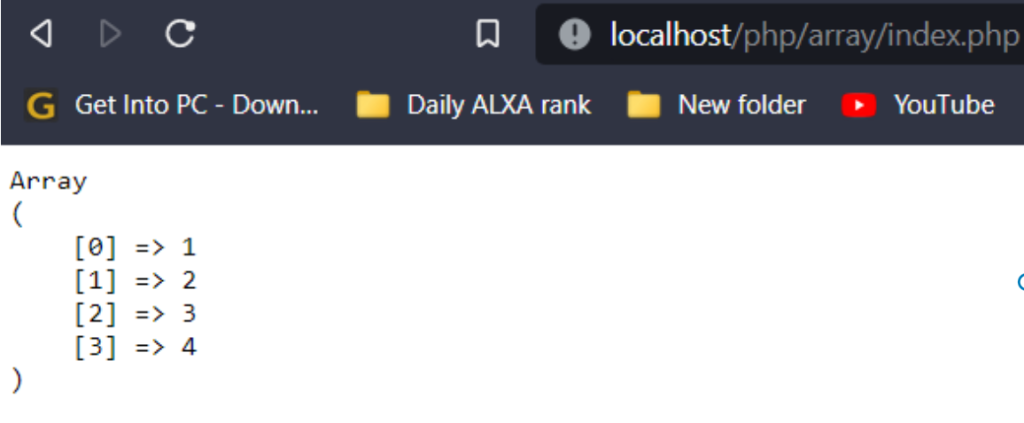
4. array_intersect() function
If you are trying to get only the common elements from two different arrays, “array_intersect” is the proper function for such a purpose.
Example:-
<?php
$myarray1 = array(1,2,3,4,-1,-2);
$myarray2 = array(-1,-2,3);
$common_array = array_intersect($myarray1,$myarray2);
echo "<pre>";
print_r($common_array);
echo "</pre>";
?>
Output:-
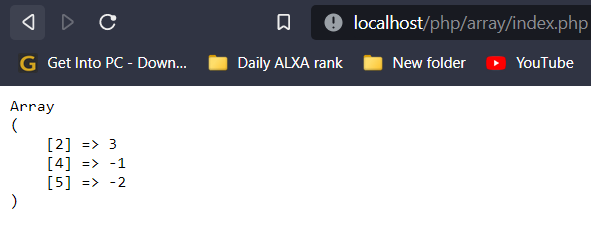
5. array_keys() function
If you somehow need to get either only the keys or values of an associative array, you can do so with help of “array_keys” or “array_values” functions.
Example:-
<?php
$my_array = array("key1"=>"value 1","key2"=>"value 2","key3"=>"value 3");
echo "<pre>";
print_r(array_keys($my_array));
echo "<br>";
print_r(array_values($my_array));
echo "</pre>";
?>
Output:-
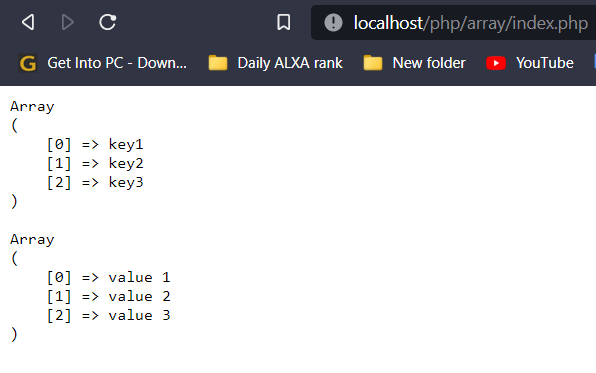
6. array_unique() function
“array_unique” – another angel function to me :D. It will remove duplicate entries and make the array contains only distinct elements. Just pass the array as its parameter and we are done! If you are trying to make unique elements that are of object type, then make sure to implement your own _toString() function inside the class and return a string value that represents the identity of the object and it should do the work fine.
Example:-
<?php
$a=array("a"=>"red","b"=>"green","c"=>"red");
echo "<pre>";
print_r(array_unique($a));
echo "</pre>";
?>
Output:-
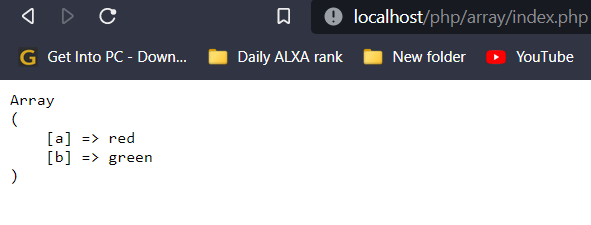
7. empty array in PHP
When an empty array and then start entering elements in it later. With the help of this, it can prevent different errors due to a faulty array. It helps to have the information of using bugged, rather than having the array. It saves time during debugging. Most of the time it may not have anything to add to the array at the point of creation.
Syntax
$emptyArray = [];
$emptyArray = array();
$emptyArray = (array) null;
Example:-
<?php
// Declare an empty array
$empty_array = array();
// Function to count array
// element and use condition
if(count($empty_array) == 0)
echo "Array is empty";
else
echo "Array is non- empty";
?>
Output:-
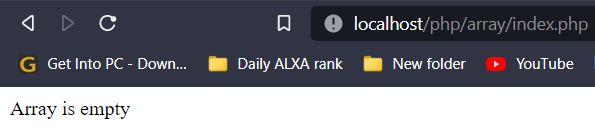
8.unset() Function
The built-in function unset()
is used to delete the value stored in a variable. It is only applicable to the local variables. It does not reflect its behavior on global variables. We can use this function to delete an element from an array.
unset($variableName);
Example:-
<?php
//Declare the array
$nicname = array("Vijay","Amit","Rahul","Dharmendra","Roshan");
unset($nicname[2]);
echo "<pre>";
echo "The array is:\n";
print_r($nicname);
echo "</pre>";
?>
Output:
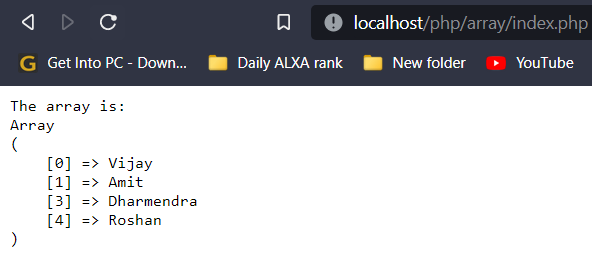
9. array_splice()
Function
The function array_splice()
is used to add elements to an array or delete elements from an array.
Example:-
<?php
//Declare the array
$nicname = array("Vijay","Amit","Dharmu","Roshan","Dilip","Rahul","Dhyanchand","Shohan");
array_splice($nicname, 4, 3);
echo "<pre>";
echo "The array is:\n";
print_r($nicname);
echo "</pre>";
?>
Output:-
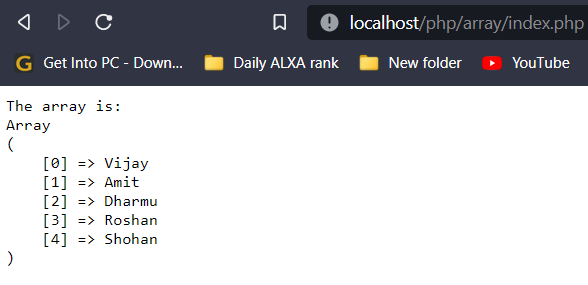
10. array_diff()
Function
The built-in function array_diff()
finds the difference between two or more arrays
. It can be used to delete multiple values from an array without affecting their indexes.
Sy
array_diff($array1, $array2, $array3, ... , $arrayN);
It takes N number of arrays
as the parameters. It compares the first array with all other arrays and returns an array that contains all the elements of the first array that are not present in other arrays.
Example
<?php
//Declare the array
$nicname = array("Vijay","Amit","Dharmu","Roshan","Rahul","Devanand","Ramayan","Dillyan");
$nicname = array_diff($nicname, array("Vijay","Amit"));
echo "<pre>";
echo "The array is:\n";
print_r($nicname);
echo "</pre>";
?>
Output:-
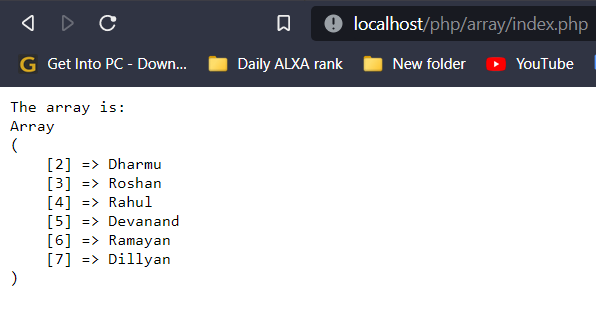
11. array_combine() function
The array_combine() function is used to combine two arrays and create a new array by using one array for keys and another array for values i.e. all elements of one array will be the keys of the new array and all elements of the second array will be the values of this new array.
Syntax:
array_combine(array1, array2)
Example:-
<?php
// Define array1 with keys
$array1 = array("subject1" ,"subject2");
// Define array2 with values
$array2 = array( "Vijay", "Ram");
// Combine two arrays
$final = array_combine($array1, $array2);
// Display merged array
echo "<pre>";
print_r($final);
echo "</pre>";
?>
Output:-
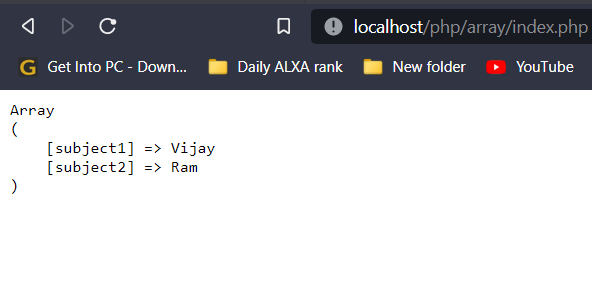
12. array_key_exists() function
The PHP array_key_exists() function checks if a specific key exists in the array. The function returns TRUE if the key is present, else it returns FALSE. array_key_exists() function works for both indexed arrays and associative arrays. For indexed arrays, an index is a key.
Syntax
array_key_exists( key, array)
Example:-
<?php
$array1 = array("a"=>"apple", "b"=>"banana", "m"=>"mango", "o"=>"orange");
$key = "m";
if (array_key_exists($key, $array1)) {
echo "Key exists in the array!";
} else {
echo "Key does not exist in the array!";
}
?>
Output:-
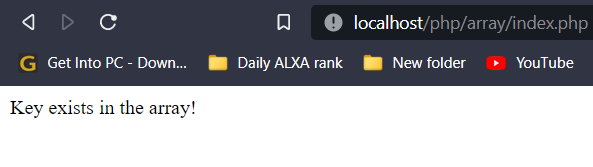
13. json_encode() function
To convert the PHP array to JSON, use the json_encode() function. The json_encode() is a built-in PHP function that converts an array to JSON. The json_encode() function returns the string containing a JSON equivalent of the value passed to it, as demonstrated by the numerically indexed array. We can also convert any JSON received from a server into JavaScript objects.
Example:-
<?php
$netflix = ['Black Mirror', '13 Reasons Why', 'Bird Box', 'Dirt'];
$netJSON = json_encode($netflix);
echo $netJSON."\n";
?>
Output:-
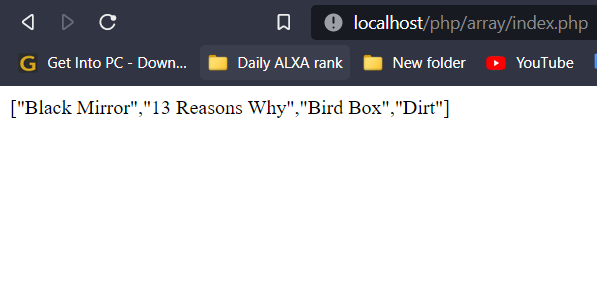
14. count()function
The count() method is used to calculate all the elements in the array or any other countable object. It can be used for both uni-dimensional as well as multi-dimensional arrays.
syntax:-
count(array)
Example
<?php
$parts = array(
"Ram" => array(
"SSD",
"Processor"
),
"Key" => array(
"Monitor"
),
"Mouse" => array(
"Cebal"
)
);
echo "<pre>";
print_r($parts);
echo "</pre>";
echo "Sub elements of an array: "
. count($parts) . "<br>";
echo "All elements of an array: "
. count($parts, 1);
?>
Output:-
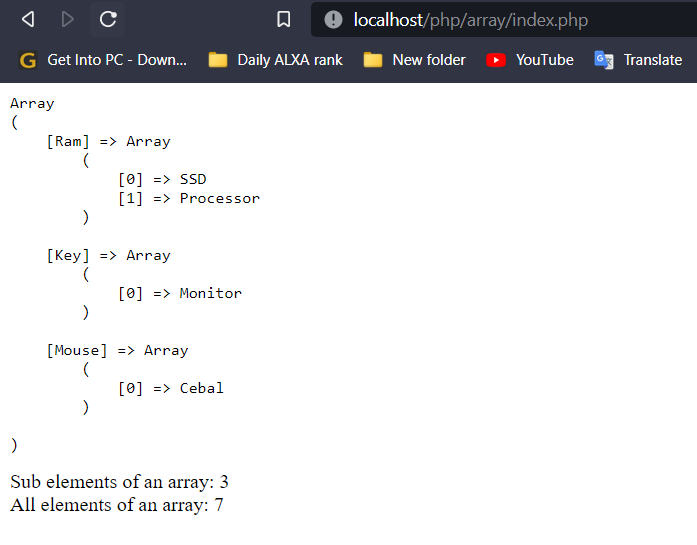
15. sizeof()
The sizeof() method is used to calculate all the elements present in an array or any other countable object. It can be used for both uni-dimensional as well as multi-dimensional arrays.
syntax
sizeof(arr, mode)
example:-
<?php
$parts = array(
"Computer" => array(
"SSD",
"Processor"
),
"Keyboard" => array(
"Monitor"
),
"Mouse" => array(
"CPU"
)
);
echo "<pre>";
print_r($parts);
echo "</pre>";
echo "Sub elements of an array: "
. sizeof($parts) . "<br>";
echo "All elements of an array: "
. sizeof($parts, 1);
?>
Output:-
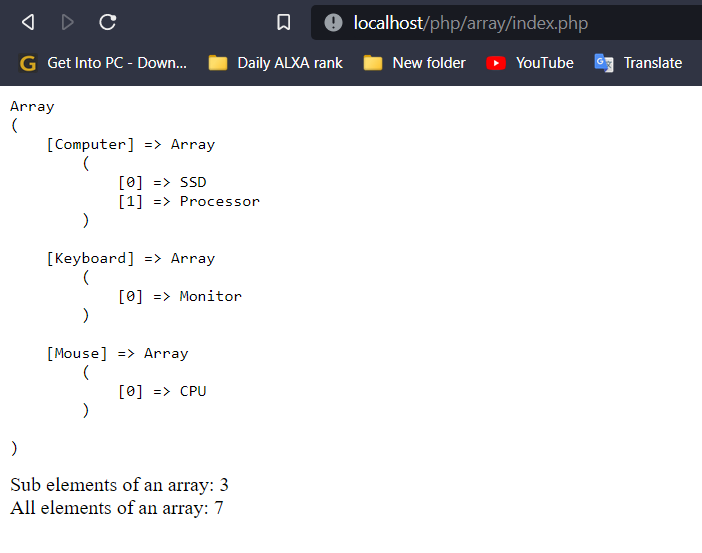
16. array_rand() function
The array_rand() function is used to get a random value from an array. This function accepts an array as a parameter and returns a random key from the array.
Syntax:-
array_rand($array, $number_of_items)
Example:-
<?php
$my_arr = ["Vijay", "Amit", "Dharmu", "Rahul", "Ravi"];
$rand_index = array_rand($my_arr);
echo $my_arr[$rand_index];
?>
Output:-
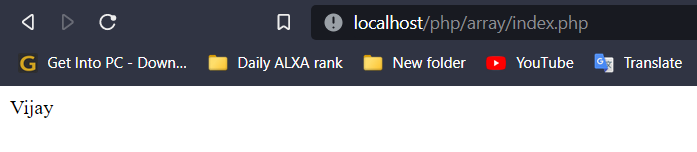
17. shuffle() function
If you’re looking for a quick and easy way to get a random item from an array using PHP, the shuffle() function is the way to go. This function will randomly shuffle the items in an array, making it easy to get a random item.
Syntax:-
shuffle($arr)[0];
Example:-
<?php
$my_arr = [
"a" => 100,
"b" => 200,
"c" => 300,
"4" => 400
];
shuffle($my_arr);
echo $my_arr[0];
?>
Output:-
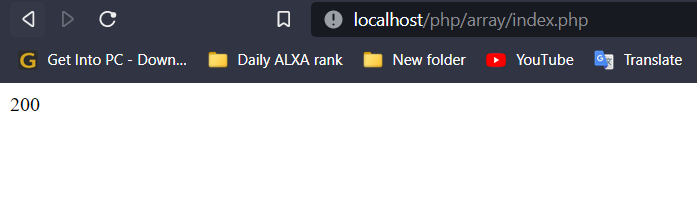
18. array_push() function
PHP array_push() is a built-in function used to insert new elements at the end of an array and get the updated array elements. The array_push() method takes a single element or an array of elements and appends it to the array.
Syntax:-
array_push(array,value1,value2...)
Example:-
<?php
$nicname = ["Vijay", "Amit", "Dharmu"];
array_push($nicname, "Ravi", "Rahul");
echo "<pre>";
print_r($nicname);
echo "</pre>";
?>
Output:-
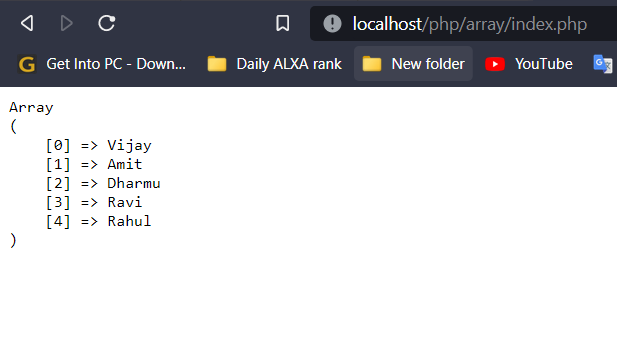
19. array_pop() function
PHP array_pop()
function to remove an element or value from the end of an array. The array_pop()
function also returns the last value of an array. However, if the array is empty (or the variable is not an array), the returned value will be NULL
.
Syntax:-
array_pop(array);
Example:-
<?php
$nicname = ["Vijay", "Amit", "Dharmu", "rahul"];
array_pop($nicname);
echo "<pre>";
print_r($nicname);
echo "</pre>";
?>
Output:-
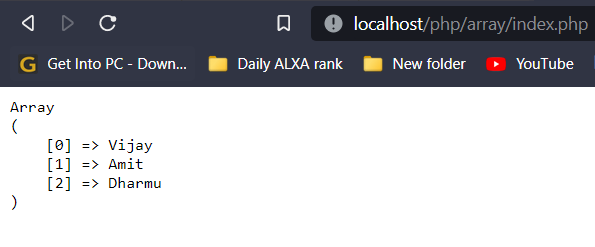
20. array_values
()
The array_values() is an inbuilt PHP function is used to get an array of values from another array that may contain key-value pairs or just values. The function creates another array where it stores all the values and by default assigns numerical keys to the values.
Syntax:
array array_values($array)
Example:-
<?php
$array = [
'phone' => 'iPhone',
'laptop' => 'Carbon X1',
'car' => 'Tesla',
];
$values = array_values($array);
echo "<pre>";
print_r($values);
echo "</pre>";
?>
Output:-
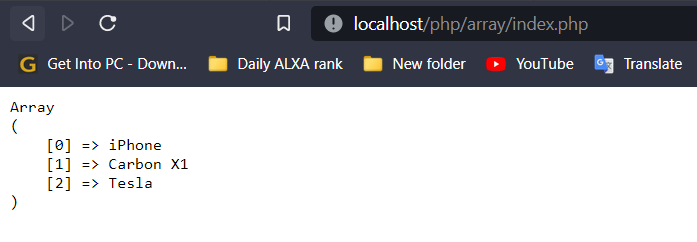