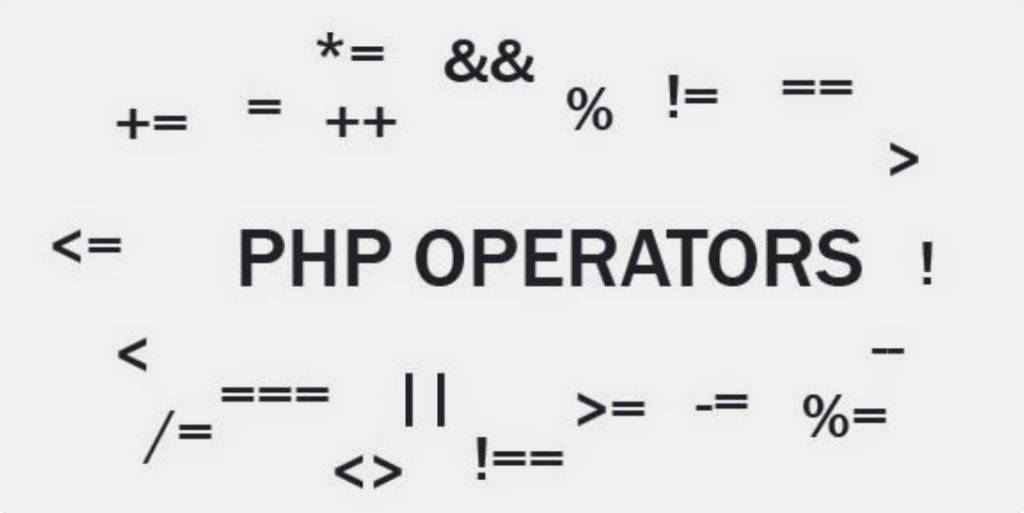
Operators are used to developing data. In Programming Languages, Operators are taken from Mathematics and Operator Programmers work with Data. An operator is a chain of symbols that is used with some values. We use Operators to perform operations on Variables and Values.
Operators help us to perform any variable or any value operation. This means that operators will give a new result by doing some operation inside any operand.
Types of PHP Operators
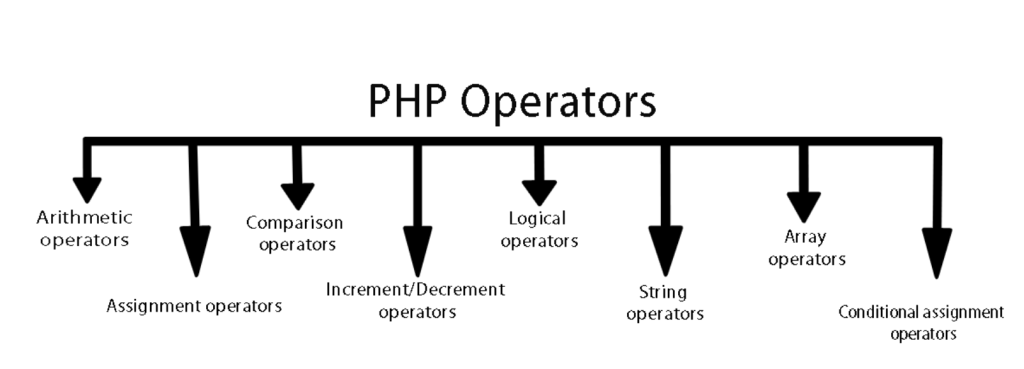
1. Arithmetic Operators
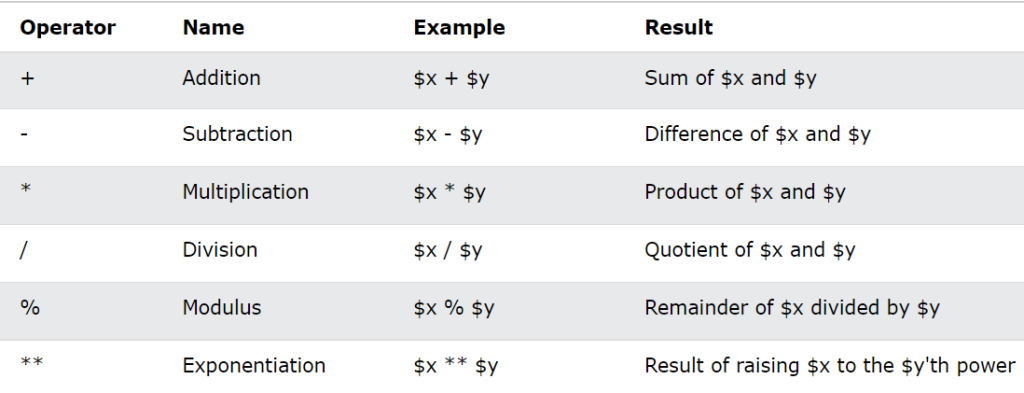
Arithmetic Operators are used to performing Arithmetic Operations with variables. These + , – , * , / , % , ++, — Arithmetic Operators are used in PHP language.
<?php
echo $a + $b . "<br>";
echo "For a + b, the result is " . ($a + $b) . "<br>";
echo "For a - b, the result is " . ($a - $b) . "<br>";
echo "For a * b, the result is " . ($a * $b) . "<br>";
echo "For a / b, the result is " . ($a / $b) . "<br>";
echo "For a % b, the result is " . ($a % $b) . "<br>";
echo "For a ** b, the result is " . ($a ** $b) . "<br>";
?>
Output:-
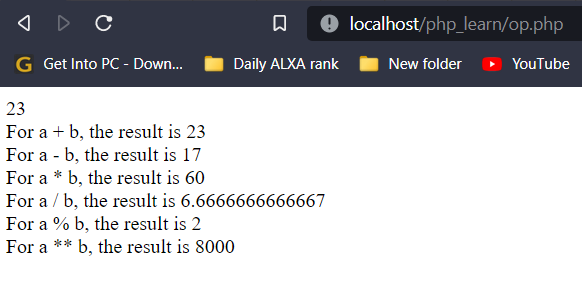
2. Assignment Operators
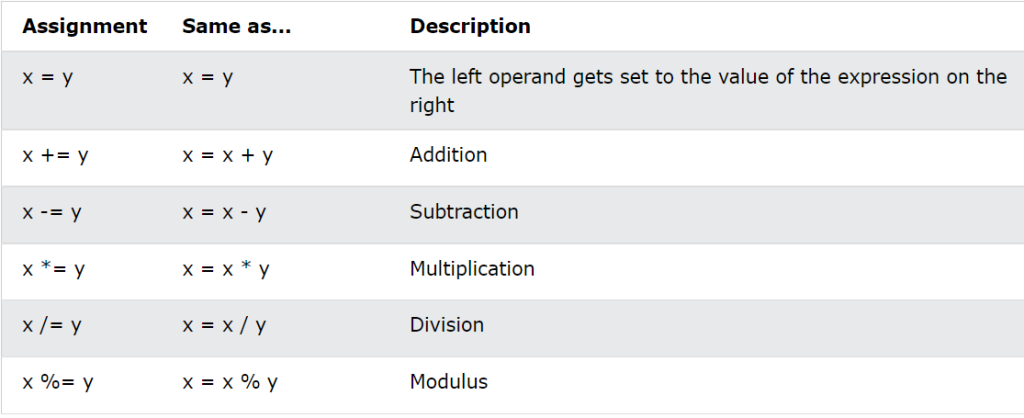
Assignment operators are used with numeric values to assign a value to a variable. In PHP language these = , += , -= , *= , /= , %= Assignment Operators are used.
<?php
$a = 20;
$b = 3;
echo "<b> Assignment Operators </b>";
echo "<br>";
echo "<br>";
$x = $a;
echo "For x, the value is ". $a . "<br>";
echo "<br>";
$a += 5;
echo "For x, the value is ". $a . "<br>";
echo "<br>";
$a -= 5;
echo "For x, the value is ". $a . "<br>";
$a *= 5;
echo "For x, the value is ". $a . "<br>";
$a /= 5;
echo "For x, the value is ". $a . "<br>";
?>
Output:-
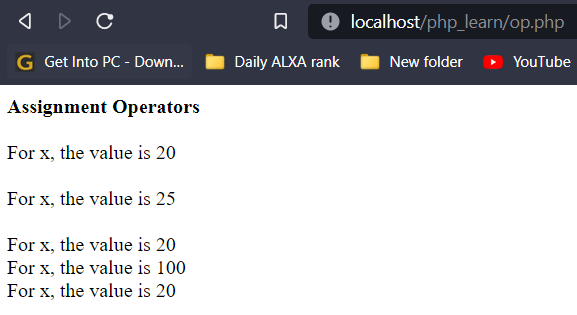
3. Comparison Operators
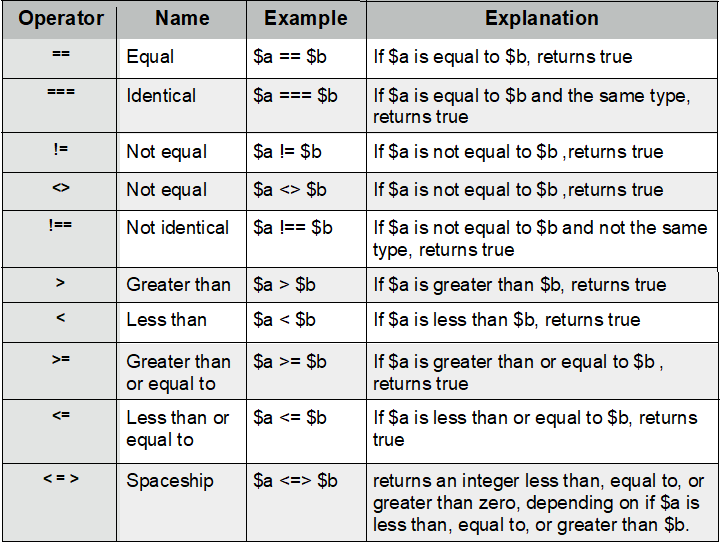
Comparison operators are used to comparing the values of two variables. These == , != , > , < , >= , <= Comparison Operators are used in the PHP language.
<?php
echo "<b> Comparison Operators </b>";
echo "<br>";
echo "<br>";
$x = 5;
$y = 7;
echo "For x == y, the result is ". ($x == $y) . "<br>";
echo "For x == y, the result is ";
echo var_dump($x == $y);
echo "<br>";
?>
Output:-
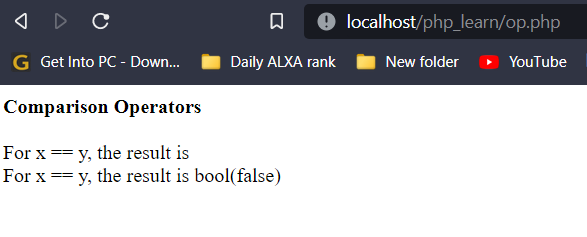
4. Logical Operators
Logical operators are used to performing logic and these operators are also used in control statements. In PHP language these and, or, &&, ||, Logical Operators are used.
<?php
echo "<b> Logical Operators </b>";
echo "<br>";
echo "<br>";
$m = true;
$n = false;
echo "For m and n, the result is";
echo var_dump($m and $n);
echo "<br>";
echo "For m or n, the result is";
echo var_dump($m or $n);
echo "<br>";
echo "For m && n, the result is";
echo var_dump($m && $n);
echo "<br>";
echo "For m || n, the result is";
echo var_dump($m || $n);
echo "<br>";
echo "For !m, the result is";
echo var_dump(!$m);
echo "<br>";
?>
Output:-
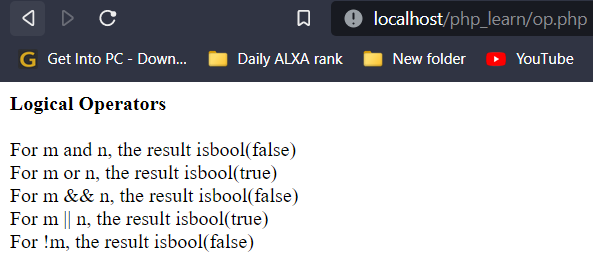