Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
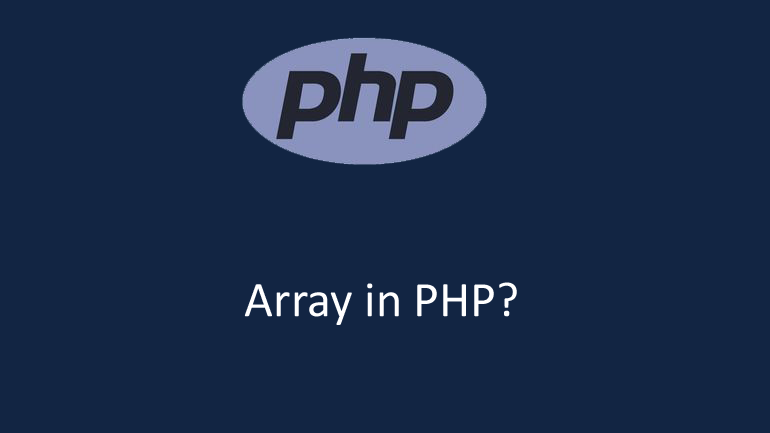
What is Array?
The array is a collection of items. The array is used for data types. The array is a special type of data structure that stores fixed-size data collection. We can store any amount of data in the array and use it easily. It also works like a database in which we can store the data. You can store it and use it. The array is a collection in which a lot of data can be stored. Array removes the complexity of the data. Many data problems can be removed by using PHP Array. The array() function is used to create an array. PHP array() function can contain (store, collect) many values.
Syntax of Array
array("value1", "value2 ", "value3");
In the above syntax, we have defined some values or items along with the array() function. PHP Array can also be defined as a database to store values. In the above syntax you can see value1, value2 and value3 in an array() function. These values(value1,value2,value3) can also be called item1,item2,item3 because array is a collection of similar items.
Examples of array
Example 1:-
<!DOCTYPE html>
<html>
<body>
<?php
$fruits = array("Banana", "Mango ", "Orange");
echo "I like " . $fruits[0] . ", " . $fruits[1] . " and " . $fruits[2] . ".";
?>
</body>
</html>
Output
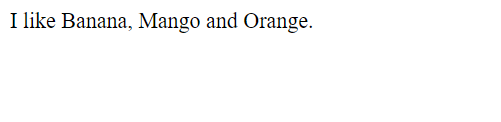
In the example above, we have created an array from a fruit variable. In Array, we have taken some items of fruits. The array contains index numbers. Index numbers start from 0 like – array(“Banana(0 index number )”, “Mango(1 index number ) “, “Orange(2 index number)”); Banana is stored at index number 0, Mango is stored at index number 1, and Orange is stored at index number 2.
Sometimes it goes out of our mind that array index numbers start from 0. If you think that array index numbers start with the number 1, then you are absolutely wrong. Keep in mind that Array index numbers start at 0. If an incorrect array index number is obtained, you will get an error or get another value. Array index numbers always start from 0.
We will also define the values one by one along with the index number of the array. This is another way to declare an array.
<?php
$x[0]=12;
$x[1]=14;
$x[2]=777;
$x[3]=44;
?>
<p>Sr No
<?php echo"$x[0]"; ?>
</p>
<p>Sr No
<?php echo "$x[1]"; ?>
</p>
<p>Sr No
<?php echo "$x[2]";
?>
</p>
Sr No <?php echo "$x[3]";
?>
Output:-
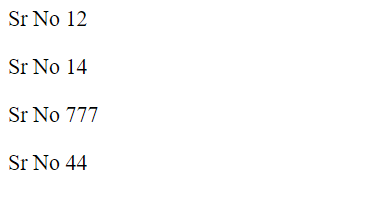
In the example above, we declared the array one after the other. $x is an array variable. In the first example, we used the variable once and we did not provide any type of index number, but in this example, we have defined different values with index numbers. So it is different from the first example.
Example 2:-
<?php
$odd_no= [1,3,5,7,9];
$first_odd_number = $odd_no[0];
$second_odd_number = $odd_no[1];
$third_odd_number = $odd_no[2];
$fourth_odd_number = $odd_no[3];
echo "This is first odd number = $first_odd_number";
echo "<br>";
echo "This is second odd number = $second_odd_number";
echo "<br>";
echo "This is third odd number = $third_odd_number";
echo "<br>";
echo "This is fourth odd number = $fourth_odd_number";
?>
Output
This is first odd number = 1 This is second odd number = 3 This is third odd number = 5 This is fourth odd number = 7
Example 3:–
<!DOCTYPE html>
<html>
<body>
<?php
$age=array("Peter"=>"35","Ben"=>"37","Joe"=>"43");
echo "Peter is " . $age['Peter'] . " years old.";
?>
</body>
</html>
Output
Peter is 35 years old.
Example 4:–
<!DOCTYPE html>
<html>
<body>
<?php
$cars=array("Volvo","BMW","Toyota");
$arrlength=count($cars);
for($x=0;$x<$arrlength;$x++)
{
echo $cars[$x];
echo "<br>";
}
?>
</body>
</html>
Output
Volvo
BMW
Toyota
Example 5:–
<!DOCTYPE html>
<html>
<body>
<?php
$age=array("Ram"=>"35","Lakshman"=>"37","Bharat"=>"43");
foreach($age as $x=>$x_value)
{
echo "Key=" . $x . ", Value=" . $x_value;
echo "<br>";
}
?>
</body>
</html>
Output
Key=Ram, Value=35
Key=Lakshman, Value=37
Key=Bharat, Value=43
Example 5:-
<!DOCTYPE html>
<html>
<body>
<?php
$color1 = "Red";
$color2 = "Green";
$color3 = "Blue";
echo $color1;
echo "<br>";
echo $color2;
echo "<br>";
echo $color3;
?>
</body>
</html>
Output
Red
Green
Blue
Example 6:-
<!DOCTYPE html>
<html>
<body>
<?php
$foo = array(1, 2, 3);
$bar = ["A", true, 123 => 5];
echo $bar[0];
echo $bar[1];
echo $bar[123];
?>
</body>
</html>
Output
A15
<!DOCTYPE html>
<html>
<body>
<?php
$arrays = [[1, 2], [3, 4], [5, 6]];
foreach ($arrays as list($a, $b)) {
$c = $a + $b;
echo($c . ', ');
}
?>
</body>
</html>
Output
3, 7, 11,
Example 6:-
<!DOCTYPE html>
<html>
<body>
<?php
$names[0] = "Rajesh";
$names[1] = "Rakesh";
$names[2] = "Abhishekh";
echo $names[1] . " and " . $names[2] . " are ". $names[0] . "'s neighbors";
?>
</body>
</html>
Output
Rakesh and Abhishekh are Rajesh’s neighbors
Example 7:-
<!DOCTYPE html>
<html>
<body>
<?php
$animals = array("Line"=>"Sher", "Cow"=>"Gai", "Tiger"=>"Bhag");
// loop through associative array and get key-value pairs
foreach($animals as $name => $value) {
echo "Name=" . $name . ", Value=" . $value;
echo "</br>";
}
?>
</body>
</html>
Outputs
Name=Line, Value=Sher
Name=Cow, Value=Gai
Name=Tiger, Value=Bhag
Example 8:-
<!DOCTYPE html>
<html>
<body>
<?php
for ($row = 0; $row < 3; $row++) {
echo "<p>Row number $row</p>";
echo "<ul>";
}
?>
</body>
</html>
Outputs
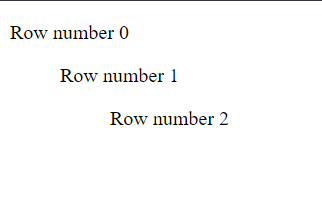
Example 9:-
<!DOCTYPE html>
<html>
<body>
<?php
$arr_variable = array (1,2,3,4,5);
foreach( $arr_variable as $arritem )
{
echo "The array item is: $arritem <br />";
}
?>
</body>
</html>
Outputs
The array item is: 1
The array item is: 2
The array item is: 3
The array item is: 4
The array item is: 5
Example 10:-
<!DOCTYPE html>
<html>
<body>
<?php
$arr = array(
"Apple",
"Banana",
"Cherry"
);
$arrString = implode(" ", $arr);
echo $arrString;
?>
</body>
</html>
Outputs
Apple Banana Cherry
We have created an array that takes odd numbers. As you know, odd numbers start with 1,3,5,7,9. An odd number means, which cannot be completely divided by 2. An odd number is divisible by the same odd number. That is, 7 is divided by 7 and 3 by 3, after that, we stored different array values ​​in different variables, and after that, we displayed array values ​​one after the other with the help of the echo() function.
Leave a Reply