Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
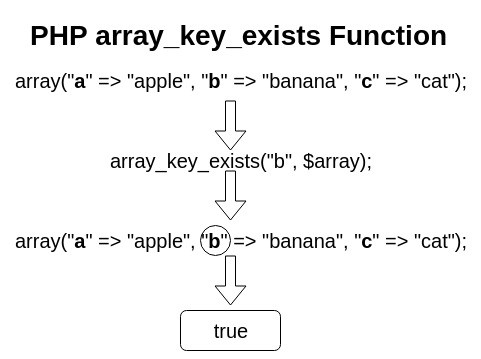
The PHP array_key_exists() function checks if a specific key exists in the array. The function returns TRUE if the key is present, else it returns FALSE. array_key_exists() function works for both indexed arrays and associative arrays. For indexed arrays, index is the key.
Syntax – array_key_exists()
array_key_exists( key, array)
Parameters
$key (mandatory)
: This parameter refers to the key/index needed to be searched for in an input array.$array (mandatory)
: This parameter refers to the original array in which we want to search the given key/indexÂ$key
.
Function Return Value
array_key_exists() returns boolean value TRUE
 if the key exists and FALSE
 if the key does not exist.
Example:-
In this example, we will take an associative array with key-value pairs, and check if a specific key "m
"
 is present in the array.
<?php
$array1 = array("a"=>"apple", "b"=>"banana", "m"=>"mango", "o"=>"orange");
$key = "m";
if (array_key_exists($key, $array1)) {
echo "Key exists in the array!";
} else {
echo "Key does not exist in the array!";
}
?>
Output:-
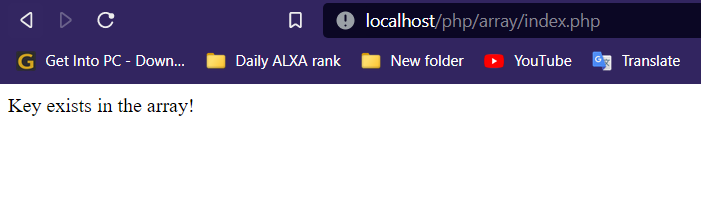
array_key_exists() returns TRUE for the given array and specified key. So, if the block is executed.
Example 2: array_key_exists() for Indexed Array
In this example, we will check if a specific index (key) is present in the array.
<?php
$array1 = array("Ram", "Sita", "Lakshman", "Bharat");
$key = "3";
if (array_key_exists($key, $array1)) {
echo "Key exists in the array True!";
} else {
echo "Key does not exist in the array False!";
}
?>
Output
The array is also printed to the console to understand the index of items in the array.
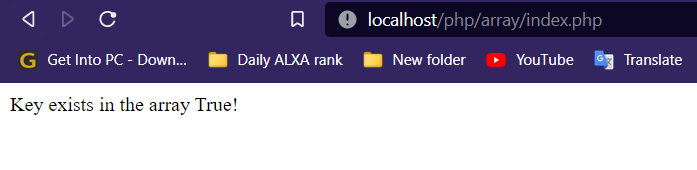
Leave a Reply