Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
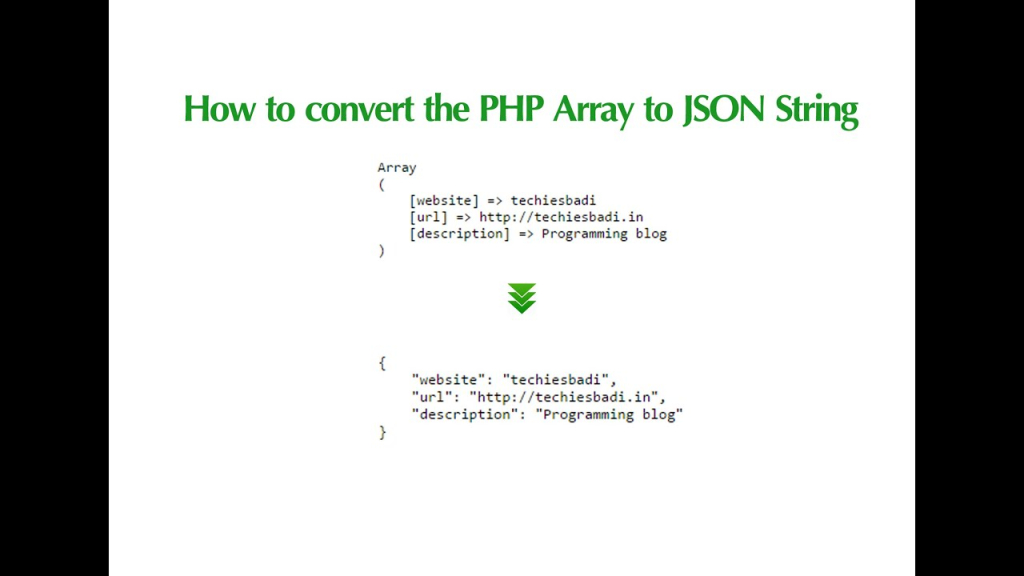
To convert the PHP array to JSON, use the json_encode() function. The json_encode() is a built-in PHP function that converts an array to json. The json_encode() function returns the string containing a JSON equivalent of the value passed to it, as demonstrated by the numerically indexed array. We can also convert any JSON received from a server into JavaScript objects.
Objects and arrays can be converted into JSON using the json_encode() method.
Example:-
<?php
$netflix = ['Black Mirror', '13 Reasons Why', 'Bird Box', 'Dirt'];
$netJSON = json_encode($netflix);
echo $netJSON."\n";
?>
Output
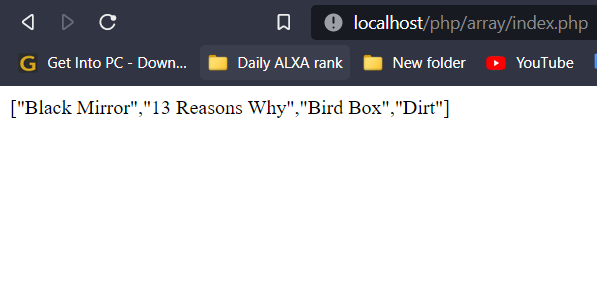
A numerically indexed PHP array is translated to the array literal in a JSON string. JSON_FORCE_OBJECT option can be used if you want that array to be output as the object instead.
Convert Associative Array to JSON
Let’s take the example of converting a key-value pair array to json.
Example:-
<?php
$data = ['name' => 'Vijay', 'blog' => 'AppDividend', 'education' => 'BE'];
$jsonData = json_encode($data);
echo $jsonData."\n";
?>
Output:-
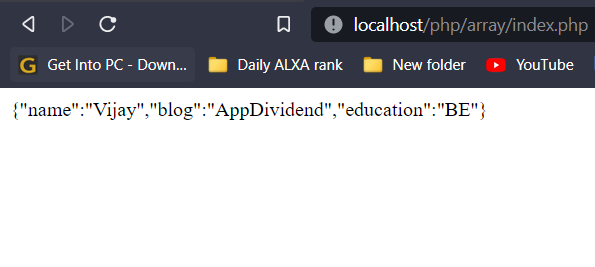
Leave a Reply