Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
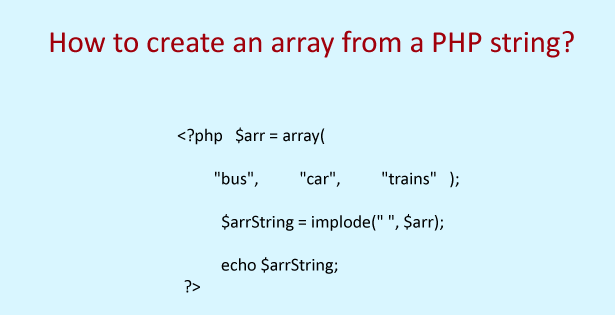
You can use the array() function with comma strings passed as an argument to it, to create an array of strings.
Syntax:-
$arr = array(
string1,
string2,
string3 );
You can use create an empty array first using the array() function and then assign strings using offset. The syntax is
$arr = array();
$arr[0] = "string1";
$arr[1] = "string2";
we will create an empty array and then assign strings as elements to it using index/offset.
<?php
$arr = array();
$arr[0] = "bus";
$arr[1] = "car";
$arr[2] = "trains";
?>
Echo String Array
You can use the echo construct and implode() function to display all elements of the string array to a browser. In the following example, we will display all elements of a string array, separated by a single space.
<?php
$arr = array(
"bus",
"car",
"trains"
);
$arrString = implode(" ", $arr);
echo $arrString;
?>
Output:-
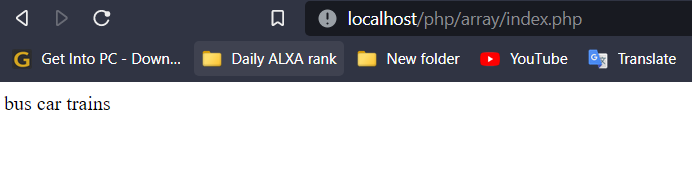
Iterate over Strings of String Array
To iterate over individual strings of a string array, you can use foreach loop, for loop, while loop, etc. In the following example, we will use a PHP foreach loop to iterate over elements of a string array.
Example:-
<?php
$arr = array(
"Maruti",
"Mahindra",
"Honda"
);
foreach ($arr as $string) {
echo " " , $string;
}
?>
Output:-
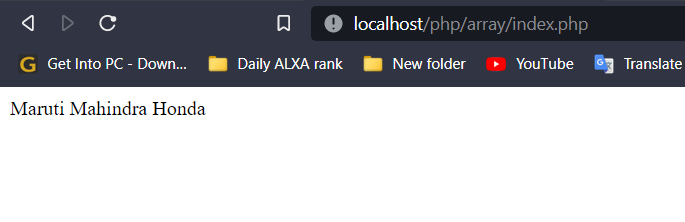
Leave a Reply