Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but won’t spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
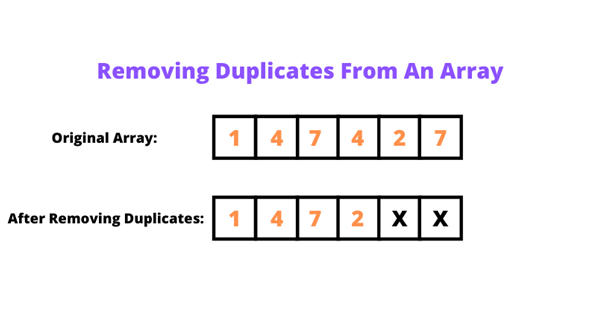
PHP array_unique
PHPÂ array_unique()
 function to remove duplicate elements or values from an array. If the array contains the string keys, then this function will keep the first key encountered for every value, and ignore all the subsequent keys.
Example:-
<?php
$array = array("a" => "One", "Tow", "One" => "Tow", "Three", "Four");
// Deleting the duplicate items
$result = array_unique($array);
print_r($result);
?>
Output:-
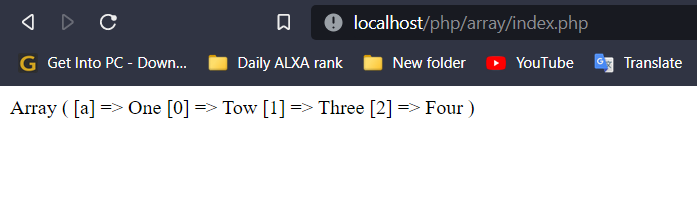
Removing duplicate values from an array in PHP
To remove duplicate values from an array in PHP, use the array_unique() function. If two or more array values are the same, the first appearance will be kept, and the other will be removed. The returned array will hold the first array item’s key type.
Syntax:-
array_unique(array, [sortingType])
Arguments
The array parameter is required, which specifies the array.
A sortingType parameter is optional, and it specifies how to compare the array element. The following are some soringType flags.
- SORT_REGULAR – compare items usually (don’t change types)
- SORT_NUMERIC – compare items numerically
- SORT_STRING – compare items as strings
- SORT_LOCAL_STRING – compare items as strings based on the current locale.
Example:-
<?php
$data = [20, 21, 21, 22, 21, 23, 22, 46, 47];
print_r(array_unique($data));
?>
Output:-
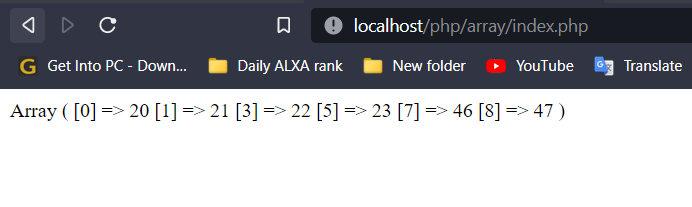
Associative Array in PHP array_unique() Function
Let’s pass the associative array to the array_unique function and see the output.
Example:-
<?php
$data = ['a' => 'Vijay',
'b' => 'Amit',
'd' => 'Vijay',
'f' => 'Amit',
'c' => 'Rahul',
'k' => 'Roshan',
'n' => 'Vijay'];
$output = array_unique($data);
print_r($output);
?>
Output:-
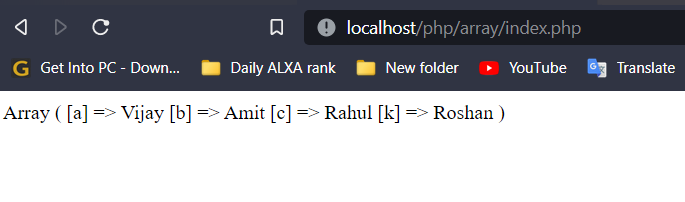
Create a multidimensional array unique
You can create a multidimensional array unique for any single key index.Â
Example:-
<?php
function unique_multi_array($array, $key) {
$temp_array = array();
$i = 0;
$key_array = array();
foreach($array as $val) {
if (!in_array($val[$key], $key_array)) {
$key_array[$i] = $val[$key];
$temp_array[$i] = $val;
}
$i++;
}
return $temp_array;
}
$data = array(
0 => array("id"=>"1", "name"=>"Vijay", "age"=>"26"),
1 => array("id"=>"2", "name"=>"Amit", "age"=>"25"),
2 => array("id"=>"1", "name"=>"Rahul", "age"=>"26"),
);
$output = unique_multi_array($data,'id');
print_r($output);
?>
Output:-

Leave a Reply